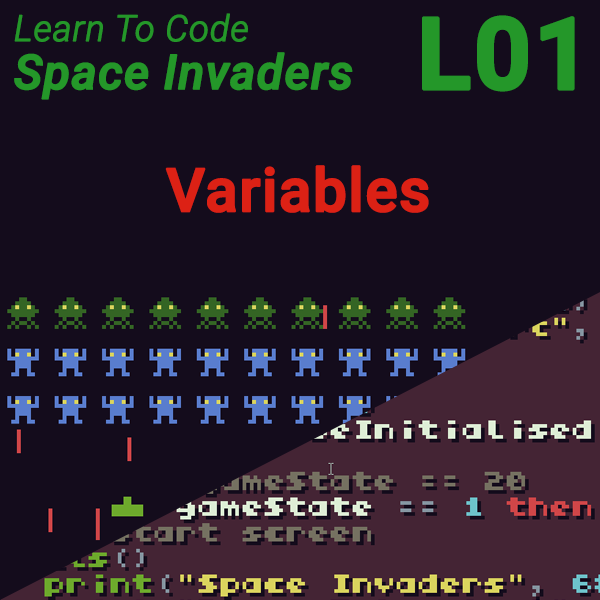
Learn to Code Space Invaders – Lesson 1 – Variables
21st July 2019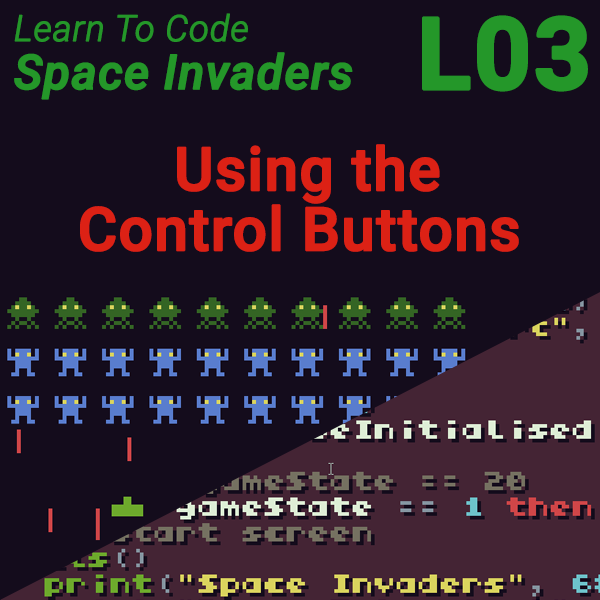
Learn to Code Space Invaders – Lesson 3 – Using the Control Buttons
22nd July 2019Space Invaders Lesson 2 – Moving Things Around
Creating Animations Using Variables
We’ve seen that variables can be used to hold pieces of information. In our code these pieces of information have a meaning. When we want to place an object on the screen we need to know its position. Most programming systems use X and Y coordinates to describe on-screen positions. These X and Y coordinates almost always start in the top left corner of the screen. As you move towards the right hand side of the screen the X coordinate increases, as you move down the screen the Y coordinate increases.
TIC 80 uses this convention. It’s screen size is 240 pixels wide by 136 pixels tall. This means that we can have X coordinate values from 0 to 239 and Y coordinate values from 0 to 135.
So, if we want to use variables to store the screen position of an object we need to use one for the X coordinate and one for the Y coordinate. So we can declare these at the start of our code.
x = 0
y = 0
These lines of code declare each variable but also assign them a value of zero. This position (0, 0) is the top left corner of the screen.
Putting Some Text on the Screen
For now we’re simply going to put some text on the screen so we can see what the values of our X and Y variables are. There’s a special command in TIC 80 to put text on the screen. We’re going to use the print statement.
print(<text goes here>, <x coordinate>, <y coordinate>)
The print statement is our first real use of what is known as a function. Functions are pieces of code that we can use to perform various tasks, in this case to put some text on the screen. There are a whole range of built-in functions in TIC 80 and will meet a number of them throughout the course. We’ll also see how we can build our own functions to perform our own tasks. But for now we just need to know how to use a function.
To use a function you simply call it by name (in this case print) followed by a set of brackets inside which we can put some information for the function to use. For the print statement the first piece of information (called a function parameter) is the actual text we want to print. This can either be a text string where we put some actual text inside some quotation marks, or a variable name which will force the print statement to use whatever text is stored in the variable. The next two parameters specify the X and Y coordinates of where on the screen you want the text to be positioned.
So if we wanted to we could use the following command to print the word hello in the top left hand corner of the screen (remember both our X and Y variables are currently set to 0).
print(“hello”, x, y)
Making the Text Move
Using variables to store the X and Y positions of our object means that we are now able to move it around the screen. Using our variables in the print statement means that it will always use the current values of our variables for the on-screen position. If our program code alters these variables then when the print command next executes, it will print the text in the new position indicated by these variables.
Going Round and Round – The Main Game Loop
For computer games to work they need to continually go through a series of operations. These can usually be grouped into updating the state of the game (moving objects, detecting explosions, etc) and drawing the game (putting everything on the screen). The Game Loop simply repeats these two sets of operations over and over as long as the game is running. TIC 80 uses a special function called TIC () as it’s game loop. All of our games have to include this TIC () function to work and we put our main control code inside it.
function TIC()
— main control code goes here
end
When we run our code this TIC () function is automatically executed 60 times per second. If our control code updates variables and then draws objects on the screen will be able to animate these objects to make them look like they’re moving around the screen.
Animating the Text
We’ve now got all the information we need to animate our text. To make a piece of text move to the right we simply need to increase its X coordinate. Since our variable X is being used to store this coordinate we simply need to increase the value of our variable.
x = x + 1
This line is assigning a new value to variable x using the assignment operator = (equals sign). The second half of the statement tells the computer what value to put into the X variable. In this case were taking the current value of the X variable and adding 1 to it. After this statement has executed our X variable will now be one bigger than it was. Adding one to a variable is called incrementing it.
If you want to move our text to the left we would need to take 1 away from our X variable, called decrementing it.
x = x – 1
Detecting When We’ve Hit the Edges – IF Statements
If we keep adding one to our X variable our text will move to the right but once our X value becomes larger than the width of the screen the text will disappear off the right hand edge. We want our text to bounce off the edge and then start moving to the left. We need a way of detecting when our X variable has become too large.
The IF statement allows our code to make decisions. It’s general format is,
if <expression that can be true or false> then
— do this
end
This is known as a conditional statement that controls program flow. The expression that the if statement uses to make its decision can be thought off as a statement that you make and if your statement is true the if statement will execute a block of code otherwise it won’t.
What Are Expressions?
Expressions can be a bit hard to get your head around. The best way to think of them is that you are stating a certain condition. The computer will then test if your condition is true or false. For example, to test if our text has hit the right hand edge of the screen we need to create a statement that describes the situation.
We know that the maximum value our X coordinate can have is 239. So when our X variable is greater than 239 will have the situation we want. So putting this into code our expression becomes
x > 239
If X had the value 200 then our expression would say
200 > 239
200 is not greater than 239 so our expression is false.
If X had the value 250 then our expression would say
250 > 239
250 is greater than 239 so our expression is now true.
If we now use this expression in our if statement it will execute its block of code only when our X variable becomes greater than 239, in other words, once it’s gone off the right hand edge of the screen.
if x > 239 then
— execute this block of code
end
Using Variables to Represent the Direction of Motion
We’ve seen that to move an object to the right or left means that we have to add one or take one away from its X coordinate. When we animate the text we not only have to remember where on the screen it is but we also have to remember which direction it’s moving. Any time we have to remember some sort of value we’ll use a variable. So our new variable will represent the X direction.
We now need to decide what values will use to represent right and left. We could store some text in the variable; “right” to move right and “left” to move left. When we come to update the value of our X coordinate we would then have to test using an if statement to see if our X direction variable contained the words right or left.
But by looking at the problem we can usually find a much easier way.
We know that when we update our X variable we need to add one to go to the right and subtract one to go to the left. So if we were to use +1 and -1 to represent right and left we could simply add our X direction variable to our X variable to update the object’s position.
x = x + xDirection
So if xDirection has the value 1 our X variable will be incremented moving the object to the right and if the xDirection variable has the value -1 our X variable will be decremented moving the object to the left.
Tests You Can Use in Your Expressions
Expressions are a big part of how our code makes decisions and appears to think. We seen the greater than operator but there are a lot more we can use as well.
Probably the most confusing one you need to learn is the equality operator, when you want to test if two things are the same. I’m purposely trying to avoid using the phrase, “are equal to each other”, simply because the symbols used can be a bit confusing.
The equality operator is a double equals sign, ==. If I want to test if variable1 has the same value as variable2 I could write,
if variable1 == variable2 then
One of the very common mistakes people make is to use the assignment operator, a single =, in their expressions.
if variable1 = variable2 then
In this line of code we’ve used the value of variable2 to assign a value to variable1. If variable2 was any value other than zero then this expression will evaluate to true, rather than actually testing if the two variables have the same value. Be very careful to use the equality operator and the assignment operator in the correct instances.
Other operators you can use are:
> greater than
< less than
>= greater than or equal to
<= less than or equal to
~= not equal to
Note ~= is the Lua symbol for not equal to. Many other languages use != or <>.
You can also combine multiple expressions using the logical operators AND, OR. If you put the AND operator between two expressions then the whole expression is true if both parts of the expression are true, i.e. expression1 AND expression2 must be true. If you use the OR operator then the whole expression is true if expression1 OR expression2 are true OR both expressions are true.
If you have very complex expressions make sure you use brackets to block test together to make sure you get the expression being evaluated in the correct order.
For example
if (x < 0) and (x > 239) then
— object has gone off the screen
end
Expanding the If Statement
So far we’ve only seen the if statement do one set of tests and execute one block of code. But it can be easily expanded to do a series of tests and choose between a number of blocks of code to execute. To do this we use both the ELSE statement and the ELSEIF statement.
if expression1 then
— do this for expression1
elseif expression2 then
— do this for expression2
else
— do this if neither expression1 or expression2 is true
end
Code for Lesson 2
-- title: Lesson2 -- author: Bob Grant -- desc: Lesson 2 -- script: lua x = 0 y = 0 xDirection = 1 yDirection = 1 function TIC() cls() x = x + xDirection y = y + yDirection if (x > 232) then x = 232 xDirection = -1 end if (x < 0) then x = 0 xDirection = 1 end if (y > 128) then y = 128 yDirection = -1 end if (y < 0) then y = 0 yDirection = 1 end print(x.." - "..y, x, y) end