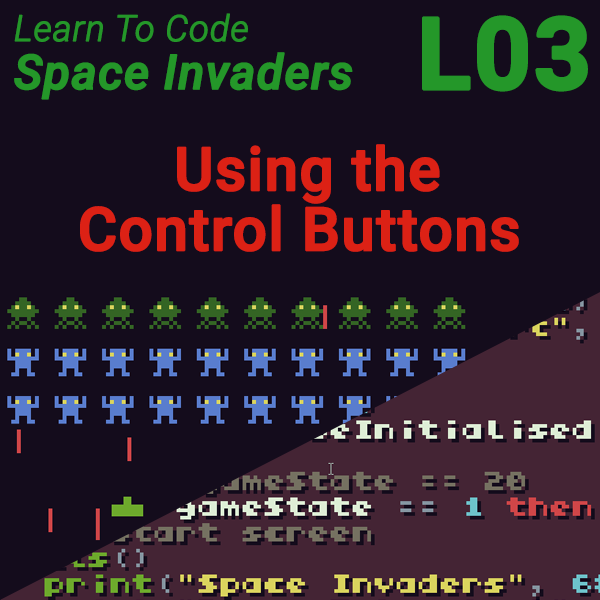
Learn to Code Space Invaders – Lesson 3 – Using the Control Buttons
22nd July 2019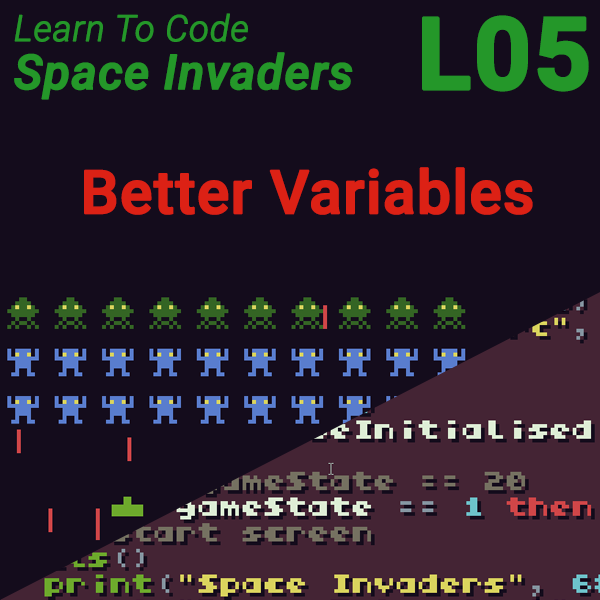
Learn to Code Space Invaders – Lesson 5 – Storing Data in Tables and Objects
23rd July 2019Space Invaders Lesson 4 – Creating and Moving Sprites
Creating and Displaying Sprites in TIC 80
TIC 80 is a complete and self-contained game development system. We’ve already been using the code editor to type in our programs, but now we need to use the sprite editor to design some images to use in our game.
You can get to the sprite editor by clicking on the second icon in the top left corner of the application window.
This will take you to the sprite editor screen which looks like this.
The sprite editor screen is really split into two halves. On the left you have the drawing area and on the right you have the preview area.
The Drawing Area
The drawing area works like a simple drawing program. You have a range of colours in a small pallet at the bottom. Above that are a number of tools you can use to draw, pick colours, select areas and so on. Above that is the actual drawing grid to the left of which is an brush size slider. Above the drawing grid is a sprite ID number.
The Preview Area
The preview area lets you see all the sprites you have currently designed. Each individual sprite is eight pixels wide by eight pixels tall. As you click on sprites in the preview area you’ll see a white box surrounding the sprite you selected which will then appear in the drawing area. The sprite ID number will also be displayed above the drawing grid.
If you need a larger sprite you can use the slider bar in the top bar above the preview area.
Putting a Sprite on the Screen
Putting a sprite on the screen is very similar to putting text on the screen. We can use another of the built-in functions to do this for us. The function we need to use is spr().
spr ( <sprite ID number>, <x coordinate>, <y coordinate> )
This is the simplest version of the function which simply needs the sprite ID number (the number above the drawing grid) and the X and Y coordinates of where you want the sprite to appear on the screen.
The spr() function is actually a lot more powerful than this. By adding a number of other parameters we can control a number of features which alter the way the sprite is displayed on the screen. The full command is shown below.
spr ( <sprite ID number>, <x coordinate>, <y coordinate>, <colour key>, <scale>, <flip>, <rotate>, <width>, <height> )
<colour key> is the index of the colour you want to be made transparent in your sprite. If you look at the pallet in the drawing area the colours are numbered from zero in the top left (usually black) to 15 in the bottom right (usually white). When the sprite is drawn on the screen this colour will become invisible so you can see what’s behind the sprite.
<scale> tells TIC 80 how many times to magnify the sprite on screen. This needs to be a whole number (an integer). For example specifying 2 will draw the sprite twice normal size.
<flip> is again an integer number that allow you to flip the sprite both horizontally and vertically. 0 specifies no flipping, 1 flips the sprite horizontally, 2 flips the sprite vertically and 3 flips the sprite both horizontally and vertically.
<rotate> is also an integer number that tells TIC 80 to rotate the sprite. You can only rotate it in 90° steps. 0 means no rotation, 1 means 90° rotation, 2 is 180° rotation, 3 is 270° rotation.
<width> and <height> specify the size of the big sprite. Big sprites are made up from a number of individual sprites. The sprite ID number is for the top left corner sprite, with the width and height parameters specifying how many sprites wide and tall to make the big sprite.
Code for Lesson 4
-- title: Space Invaders -- author: Bob Grant -- desc: Lesson 4 -- script: lua playerShipX = 120 playerShipY = 128 function TIC() cls() if(btn(2)) then playerShipX = playerShipX - 1 end if(btn(3)) then playerShipX = playerShipX + 1 end if (playerShipX < 0) then playerShipX = 0 end if (playerShipX > 232) then playerShipX = 232 end spr(0, playerShipX, playerShipY) end