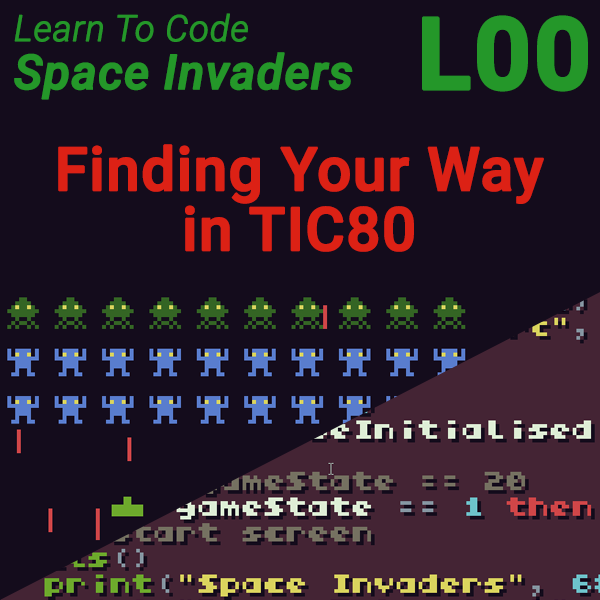
Learn to Code Space Invaders – Lesson 0 – Finding Your Way in TIC80
20th July 2019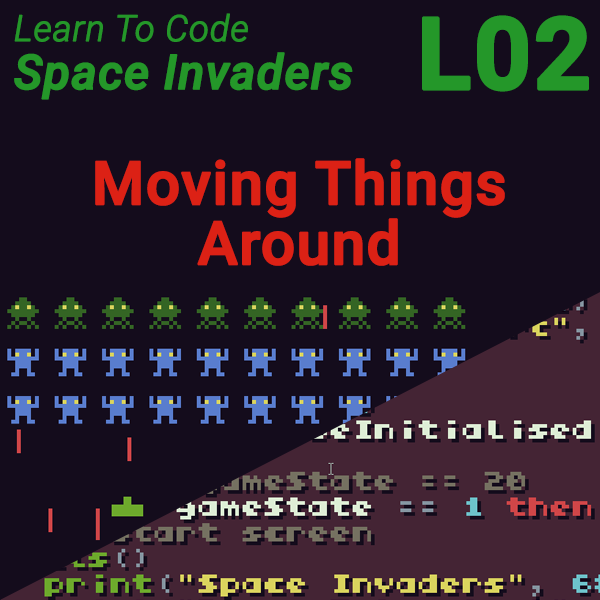
Learn to Code Space Invaders – Lesson 2 – Moving Things Around
22nd July 2019Space Invaders Lesson 1 – Variables
Getting Your Program to Remember Things Using Variables
All computer programs need to remember bits of information. This data could be the position of a missile on the screen, the player’s current score, how many lives the player has left or any number of other details. The simplest way for your software to store this information is to use a variable.
Variables are like labelled boxes you can put a piece of information into. When you create a variable you have to give it a name (the label on the box) and the computer will allocate a small amount of storage space in its memory (the box) to hold your information. When you put some information into the variable, called assigning a value to it, the computer (or whatever device your code is running on) will copy that information into the storage space it allocated for your variable. When you want to use the variable the computer will have a look at what information is stored in the allocated space and make that data available to your code.
The best way to see how variables work is to look at a short piece of code.
myVariable = 10
doubleVariable = myVariable * 2
(asterisk * means multiply)
The first line of code creates the variable (called declaring the variable) and stores the number 10 inside it (assigns the value 10 to the variable).
The second line declares a second variable and again assigns a value to it. When we use the first variable, myVariable, in this assignment the computer has a look at what stored inside the variable and places that into the code where the variable name is being used. So, our second line of code becomes
doubleVariable = 10 * 2
At the end of this code are software will have two variables. One called myVariable with the number 10 stored inside it and another called doubleVariable with the number 20 stored inside it.
Our code could then continue to reassign values to our variables, for example
myVariable = 100
would then change the contents of myVariable to 100.
Naming Variables
There are a number of rules for naming variables. Variable names must :
- only contain letters (upper and lower case), numbers and underscores (_)
- must not begin with a number
- must not be the same as a language keyword
Different people use different formats for names. I tend to use camel case in the lessons. As you’re not allowed to use spaces you can break up words using capital letters, e.g. thisIsTheVariableName. Some people prefer underscores, e.g. this_is_the_variable_name. The choice is really up to you but make sure you stick to one way of writing the names.
Some languages, and some companies you might work for may specify a naming convention (the way you write variable names).
The name you choose should describe what information the variable stores. It’s tempting to make the names single letters or some random words, but naming variables correctly really makes your code much easier to debug and understand. You’ll also find that giving your variables descriptive names makes your code also read like a normal sentence, e.g.
numberOfAliens = aliensPerRow * numberOfAlienRows
Variable Types – What Can You Store in a Variable?
When we talk about variables we need to consider what types of information they hold. In the above examples we were storing whole numbers. In computer terms these are called integers, so the two variables we created were integer variables. We could have stored a decimal number in our variable
myVariable = 5.4
This would have created a floating point or real number variable. We could also have stored a piece of text.
myVariable = “Some text in the variable”
Note that we have to put the text inside quotation marks so that our software understands that this is text and not some program code. When we put text into a variable it’s called a string variable as it holds a string of text.
We can also have variables that can only have the values true or false. These are called Boolean variables and can be used to flag when certain events or conditions occur in our software.
The last main type we need to consider is a character variable. These store a single text character such as the letter A, etc.
There are a number of other types of variables but we’ll meet those later in the course.
Summary
You need to declare a variable before you can use it. To do this simply assign a value to the variable name.
myVariable = 20
When you use a variable name in your code the software will replace that variable name by the value contained inside it.
newVariable = myVariable + 10
becomes
newVariable = 20 + 10
So newVariable has the value 30.
Code for Lesson 1
-- title: Lesson1 -- author: Bob Grant -- desc: Lesson 1 -- script: lua count = 0 double = 0 x = 100 y = 50 function TIC() cls() count = count + 1 double = count * 2 print(count, x, y) print(double, x, y + 8) end