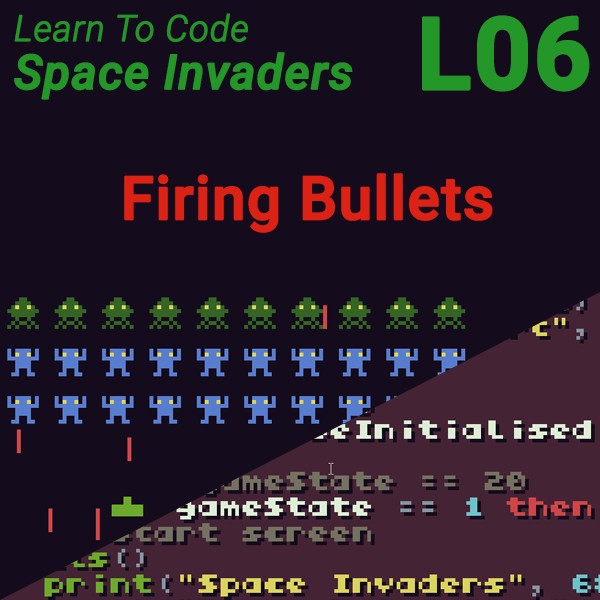
Learn to Code Space Invaders – Lesson 6 – Firing Bullets
23rd July 2019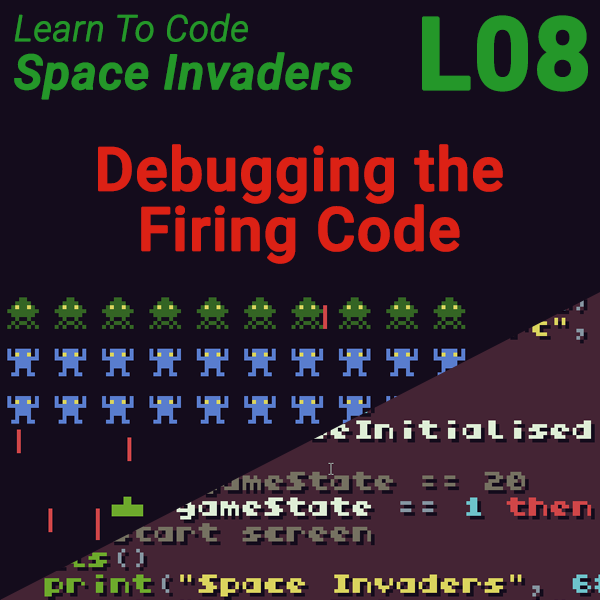
Learn to Code Space Invaders – Lesson 8 – Debugging the Firing Code
30th July 2019Space Invaders Lesson 7 – Functions
Breaking Our Code up Into Functions
As we add more features to our program our code is starting to get longer and longer. At the moment we are just adding our new code onto the end of our existing code. If we continue doing this we’ll end up with a single, very long block of code that performs all of the operations needed for our game. Whilst this style of programming can still produce a working game it makes it harder for other people, and indeed ourselves, to understand how the software works. We end up with a tangled mess of program statements that have been created in the order we thought them up rather than any structured layout.
This is why we always need to think about breaking our software up into manageable and understandable parts. Each of these parts will perform a very specific operation and when we put all the parts together we’ll have our full game.
Functions to the Rescue
Functions are the first method we’ll use to break up our code.
Functions are simply small pieces of code that we can give a name to. We can also pass some information to our function as parameters and if required the function can return some information for us to use.
Using functions provides us with two main advantages.
They help us break up and structure our code to make it much more easy to understand and to fix problems in the future (debugging and modifying our code).
Once the function has been defined it can be used in multiple places within our code. We only have to write the function once and then call it, rather than having to type in the code everywhere we need to use it. Having multiple versions of the same code is almost always a bad idea. If you ever have to modify the code you then have to make sure that you modify all versions of it. With a function you simply modify the function definition and then everywhere it’s used will automatically be updated.
Creating Functions
To create function we simply use the function keyword followed by the function name. We then have to use a set of brackets which can either be empty if the function doesn’t require any information, or we can add variable names, called the functions parameters, to capture any information we want to send to it.
The actual code for the function is usually indented a little bit so that we can see which lines are part of the function body.
In Lua we signal the end of the function by using the end keyword.
So our general layout for defining a function is:
function myFunction() -- function code goes in here end
To use a function in our code (we refer to this as calling the function) we simply call it by name.
myFunction() -- this calls the function
When we call function it’s as if we had typed the code for the function in that place. For example.
playerScore = 0 -- define the player score variable function drawScore() -- define the function print(playerScore, 0, 0) -- print the score on the screen end -- end the function definition function TIC() -- our main game loop function drawScore() -- call the function to print the score end -- end of our main game loop
Naming Functions
It’s important to give our functions meaningful names that describe what they do. If a function handles the moving of the player ship then we should give it a name like movePlayerShip. This makes it easy for us to remember what the function does, but also makes the code where we use the function almost readable language. For example our main game loop will eventually be a list of function calls.
function TIC() movePlayerShip() moveAliens() movePlayerBullets() drawPlayerShip() drawAliens() drawPlayerBullets() end
Even now, even though we haven’t written any of the functions, the code should make sense and you should be able to understand how the software is going to work as it steps through the stages of the game. We don’t know how to move the aliens, but we know that we’ll create a piece of code to do it and that that piece of code will be called as part of our main game loop.
Function names obey the same rules as variable names.
- only use letters, numbers and underscores (_)
- names must start with a letter
- don’t use reserved language keywords, e.g. while, for, etc.
Sending Information to Our Function – Parameters
If we need to send some information to our function we can add parameters between the brackets on the function declaration line. These parameters become variables that we can use inside our function code.
function myFunction(parameter1)
This line creates a function called myFunction that expects to be sent one piece of information. That piece of information will be put inside a variable called parameter1 which we can then use inside our function’s code. This is what’s known as a local variable (we’ll talk more about this later) which means that once the function has finished the variable will be deleted so that it won’t be available elsewhere in our code. If we call the function a second time the local variable will be recreated to capture the new piece of information being sent to the function.
So if you wanted to send the number 10 to our function we would call it like this:
myFunction(10)
The value 10 would be caught by parameter1. So in our function code the local variable parameter1 will automatically be initialised with the value 10.
We can send as many parameters as we want, we simply separate them using commas in our function declaration.
function myFunction(parameter1, parameter2, parameter3)
And we can call this function and give it three values using:
myFunction(10, 20, 30)
Getting an Answer Back from a Function – Return Values
Sometimes our functions need to send back an answer. We do this using a return value. This return value can be anything we want, an actual number, a string of text or if we return a variable, then we will return the value contained within that variable.
For example we could write a function that where we send a single number as a parameter and have it return back that number doubled.
function doubleNumber(number) answer = number * 2 return answer end
We can then use this function in our code to calculate the double of any number we want. As the function is returning some information we need to catch it using a variable. This is done by simply assigning our variable the value returned by our function. For example:
singleNumber = 20 doubled = doubleNumber(singleNumber)
In this code we first create a variable called singleNumber and assign it the value 20. We then create a second variable called double and assign it the value returned by calling our doubleNumber function using our first variable to pass the value 20 as a parameter.
At the end of this code the variable doubled will have the value 40.
Functions, Procedures and Subroutines
There are a number of different names we use to describe functions. Most programming languages use the function key word and most programmers will talk simply about functions. But sometimes, especially in computer science examinations, you will hear procedures and subroutines mentioned.
Strictly speaking a procedure is a named block of code that simply performs a set of operations. It can accept a number of parameters but it doesn’t return back a value.
A function is the same as a procedure, but it will always return a value.
Subroutines are basically the same as procedures, i.e. they don’t return back a value.
Keeping Our Code Well Structured
From now on as we develop our space invaders game we’ll be breaking up all of our code into functions. Each function will handle a specific part of our game code to keep it well organised and more easily understandable.
Code for the Functions Test Program
-- script: lua a = 5 b = 3 biggest = 0 function TIC() cls() print("a = "..a..", b = "..b, 0,0) print("biggest = "..biggest, 0,8) end