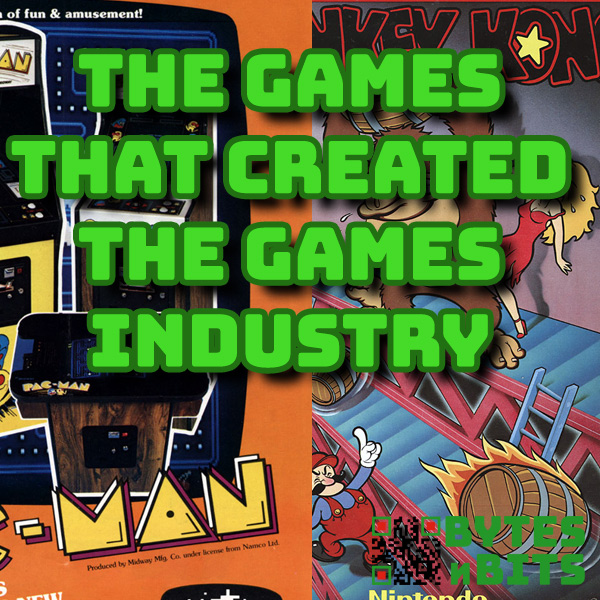
The Games That Created the Gaming Industry – The Golden Age of Video Games
16th June 2021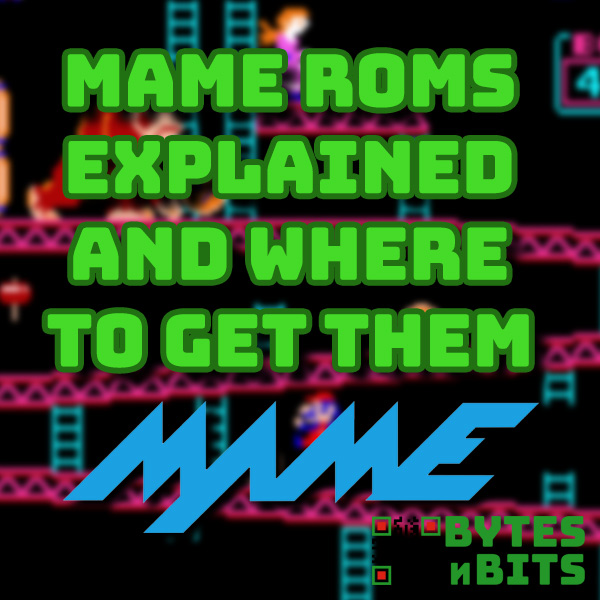
MAME ROMs Explained and Where To Download Them
19th July 2021Modelling the Game World in Space Commander – Learn to Code Your Own Games
The Space Commander world is modelled as a 2D space, like looking at a map from above. Objects in the game exist and move in this 2D space. All our calculations will take place in this game world. When it’s time to draw objects on the screen we’ll then map the game world to our screen world.
To describe objects’ position and motion we need a few parameters.
Position
This is the (x, y) coordinates of the object in the 2D game space. Screen coordinates tend to use the top left corner as the (0, 0) position with the positive x axis to the right and the positive y axis to the bottom. This differs from the conventional mathematical axes which have the positive x axis to the right but the positive y axis to the top.
It’s really a personal choice but I usually stick to the mathematical model unless the game world is quite simple. In this case we’ll be modelling vector quantities for velocity and acceleration, creating targeting code, etc., so using the mathematical model just makes it easier (for me) to see how angles and positions fit together.
You can make the game world match the screen world, but it doesn’t save much computing power and I can never get my head around the flipped maths!
Direction
When objects move or rotate, we need a way to describe their direction, or the way they are pointing. Using normal mathematical notation this gives us a 0 degree angle parallel to the positive x axis (i.e. horizontally to the right) with positive angles measured in an anti-clockwise direction from it.
Using this notation makes all our angle, sine, cosine and tangent calculations match with what we would expect.
Distance
As our world is imaginary, we don’t have to worry about real world distance measurements. Our positions will be easier if we use pixel coordinates, so it makes sense to measure distances in pixels as well.
Time
Again, we get to choose our units of time. We can use seconds, but a more natural measurement inside the code is the game loop. Using one game loop as one unit of time simplifies the whole time process. If we set a speed of 5 pixels per loop, we simply need to add 5 pixels each time our game loop runs.
This method can have problems. It relies on the game loop running at a set speed. TIC80 runs the game loop at 60 loop per second. If our code gets too complex each game loop takes longer than 1/60 second to complete. Our game will start to slow down, and objects will visibly move slower on the screen.
If you’ve played any retro computer games, you’ll have seen this in action.
Making the World Infinite
We want the player to be able to fly as far as they want in any direction, giving this idea of an infinite playing space. If we use a standard position, measured in pixels from a fixed origin we’ll hit a problem.
In computers, numbers have limits. If we use an integer variable to model the x coordinate of the player there will be a maximum (and minimum) value that this variable can store.
If you’re familiar with C programming, you’ll understand about using different numbers of bytes to store integers. A single byte (8 bit) integer can store values from 0 to 255. A 16-bit integer can store 0 to 65535. And so on.
If our player were to fly in one direction for a long time the coordinates may reach the limits of our variable. E.g. using 8-bit integers we can count up to 255, but adding 1 to this will result in an overflow error and our variable will wrap round to 0.
To get around this potential problem we’ll use the fact that we always want to player to be in the centre of the play area. So, we’ll use the player ship as our origin and have everything moving relative to it. So, if the player ship is travelling up, we keep the player stationary, but move everything else down, giving the same end movement.
In TIC80, which uses Lua, all numbers are represented by 64-bit floating-point numbers (real numbers with decimal places!). 10 bits are used for the exponent (the power of ten), 1 for the sign (positive and negative) and 53 for the mantissa (the significant figures of the number). This allows Lua to represent integer values from -2^53 to +2^53. We’d never reach that value, but I hope you get the idea of what we’re doing. If you ever do code in C, or even worse assembly, you’ll now know what to do!
Modelling the position and Movement of Objects
So now we know the basic parameters we’re using let’s look at how we’ll model our data.
We want to create several helper functions that will allow us to calculate positions, movement, rotations and so on. We’ll have a few different object types for our player ship, enemy ships, missiles, stars, etc.
We could write separate functions for each object to, for example, rotate it. But each of these functions would basically be the same code repeated multiple times and this isn’t good coding practice.
So, we want each operation to be handled by a single helper function which will be able to work with all our objects.
e.g. rotate_object(player_ship) and rotate_object(enemy_ship) should both work.
This relies on each object in our game storing its model data in the same format. So, when we create a class (object definition) for each game object it will inherit this data format. This is one of the principles of Object Orientated Programming (OOP) – Inheritance. Or we can think of each object providing a defined set up to its values, an OOP Interface.
If you’re interested in learning Object Orientated Programming, please check out my Python courses on Udemy.
In TIC80 we have to implement this manually, but in other languages, such as Python, we can do it through the OOP coding system.
So, each object in the game will have,
- a position attribute with x and y coordinates.
- a velocity attribute with an angle and magnitude
Moving Objects in the 2D World
The Player Ship
We’ve already seen that the player ship isn’t going to move. Its position will always be at (0, 0). But it will have a velocity with angle and magnitude.
To move the player ship, we work out its x and y position increments, dx and dy.
dx = velocity.magnitude x cos(velocity.angle) dy = velocity.magnitude x sin(velocity.angle)
We then take these away from the positions of every other object in the game.
for every_other_object position.x = position.x - dx position.y = position.y - dy next every_other_object
Every Other Object
Because we’re modelling our velocities as vectors (magnitude and direction) we can simply add our movements together to get the resultant motion. Each object has its own position and velocity, so we again calculate the incremental movement due to that motion and simply add that to its position after (or before) the movement relative to the player ship.
move object relative to player ship dx_object = velocity.magnitude x cos(velocity.angle) dy_object = velocity.magnitude x sin(velocity.angle) object.position.x = object.position.x + dx_object object.position.y = object.position.y + dy_object
This then gives the new object position in (x, y) format using the player ship as the (0, 0) origin.
Mapping the Game World to the Screen
So, all of the previous calculations allow us to move our objects around in the 2D game world where we are obeying all the mathematical norms for the axes and angles. We need to work out how to draw this on the screen.
We know that we always want the player ship pointing vertically. This is a 90 degree angle, measured from the positive x axis. If the player ship is currently travelling at an angle A, we would need to rotate the whole game map by (90 – A) degrees to get the ship pointing up the screen.
Our screen coordinates are measured from the top left corner, with x increasing to the right and y increasing down the screen. So, to map our 2D game world to the screen we need to take the game world x coordinate, but the negative y coordinate. We then need to offset this by half the screen width and half the screen height to move the origin to the centre of the screen.
So, our helper function will look like,
new_position(x,y) = rotate (game_world_position around origin by (90 - player_ship_angle) degrees) new_position.x = new_position.x + screen_offset_x new_position.y = -new_position.y + screen_offset_y
This leaves new position with the screen coordinates version of the game world position.
Mapping Object Rotation to the Screen World
As we rotate an object around the origin to position it on the screen its coordinates will change, but so will its angle of rotation.
We know that we need to rotate the game world by (90 – player_ship_angle). So, when we draw an object on the screen world its rotation will also increase by the same amount,
object_screen_angle = object_game_world_angle + (90 - A)
Coding the World Display
So, we’ve now got all the information and calculations we need to code this game world and map it to the screen.
Please have a look at the video and the code below to see how I’ve implemented our algorithms.
Play the Demo Code
Code
-- title: Space Commander -- author: Bob Grant -- desc: -- script: lua -- global variables -- game states state={} state.start_screen = 0 state.playing = 1 state.player_exploding = 2 player_exploding_time = 300 state.level_up = 3 player_level_up_time = 300 state.end_game = 10 player_end_game_time = 400 state.scoreboard = 20 frame_timer = 0 -- TIC80 screen 240 x 136 screen_max_x = 239 screen_max_y = 136 screen_offset = {x=119, y=77} player_ship_max_bullets = 6 player_ship_rotation_speed = 0.015 player_ship_max_velocity = 1.5 player_ship_acceleration = 0.05 player_ship_radius = 7 player_ship_max_health = 50 player_bullet_velocity = player_ship_max_velocity * 2 player_bullet_max_age = 60 player_bullet_damage = 1 player_ship_collide_damage = 25 player_max_lives = 3 max_player_level = 4 max_enemies = 3 total_enemies = 3 enemy_id_counter = 0 enemy_ship_max_bullets = 6 enemy_ship_rotation_speed = 0.02 enemy_ship_max_velocity = 1.5 enemy_ship_radius = 4 enemy_max_health = 5 enemy_acceleration = 0.05 enemy_bullet_velocity = enemy_ship_max_velocity * 1.5 enemy_bullet_max_age = 60 enemy_bullet_damage = 1 enemy_bullet_reload = 150 enemy_target_distance = 70 enemy_shooting_range = 80 enemy_rebound_angle = math.pi enemy_rebound_speed = 1.0 enemy_min_visible_dist = 100 enemy_ship_respawn_dist = 2000 max_enemy_ship_type = 4 background_max_objects = 20 debug_value1=0 player_ship = {} player_bullets = {} enemy_bullets = {} enemies = {} particles={} background={} function init_variables(reset_enemies) -- player ship player_ship = {} player_ship.ship_type=player_level player_ship.position = {x=0, y=0} player_ship.velocity = {magnitude=0, angle=(math.pi/2)} player_ship.movement = {dx=0, dy=0} player_ship.radius = player_ship_radius player_ship.health = player_ship_max_health player_ship.timer = 0 if(reset_enemies) then -- respawn enemies enemies = {} max_enemies = (5*player_level) + 5 total_enemies = max_enemies * 3 enemy_id_counter = 0 else -- keep existing enemies but move them a bit further away for index,ship in ipairs(enemies) do ship.position.x = ship.position.x *1.5 ship.position.y = ship.position.y *1.5 ship.velocity.magnitude = 0 end -- for end particles={} background={} spawn_background{} end -- init_variables function init_game_state() game_state = state.start_screen last_game_state = game_state player_lives = player_max_lives player_score = 0 player_level = 0 end -- init_game_state ship_angle = 0 first_run = true function TIC() if first_run then for counter = 1,4 do spawn_enemy_test(counter, -100 +(40 * counter),50) end -- for first_run = false else cls() update_movement_params() update_background() check_ship_rotation() check_ship_acceleration() move_enemy_ships() draw_background() draw_player_ship() draw_enemy_ships() end -- if end -- TIC function spawn_enemy_test(test_ship_type, x,y) local enemy_ship = {} enemy_ship.ship_type = test_ship_type local spawn_angle = 0 local ship_x=x local ship_y=y enemy_ship.position = {x=ship_x, y=ship_y} enemy_ship.velocity = {magnitude=0, angle=0} enemy_ship.hit = false enemy_ship.movement_type=0 enemy_ship.timer = 0 if(enemy_ship.ship_type == 1) then enemy_ship.rotation_speed = 0.02 enemy_ship.max_velocity = 1.5 enemy_ship.max_health = 5 enemy_ship.health = 5 enemy_ship.acceleration = 0.05 enemy_ship.target_accuracy =0.2 enemy_ship.bullet_velocity = enemy_ship.max_velocity * 1.5 enemy_ship.bullet_max_age = 120 enemy_ship.bullet_damage = 1 enemy_ship.bullet_tracking = false enemy_ship.bullet_rotation_speed = 0.007 enemy_ship.bullet_trail = false enemy_ship.fire_speed = 32 enemy_ship.bullet_reload = 150 enemy_ship.bullets = 0 enemy_ship.bullet_radius = 4 enemy_ship.abs_max_bullets = 6 enemy_ship.target_distance = 50 enemy_ship.shooting_range = 80 enemy_ship.radius = 5 enemy_ship.score = 50 enemy_ship.bullets = 0 enemy_ship.max_bullets = math.floor(math.random(enemy_ship.abs_max_bullets)) + 1 elseif(enemy_ship.ship_type == 2) then enemy_ship.rotation_speed = 0.03 enemy_ship.max_velocity = 1.7 enemy_ship.max_health = 5 enemy_ship.health = 5 enemy_ship.acceleration = 0.1 enemy_ship.target_accuracy =0.15 enemy_ship.bullet_velocity = enemy_ship.max_velocity * 1 enemy_ship.bullet_max_age = 180 enemy_ship.bullet_damage = 2 enemy_ship.bullet_tracking = true enemy_ship.bullet_trail = true enemy_ship.bullet_rotation_speed = 0.01 enemy_ship.fire_speed = 32 enemy_ship.bullet_reload = 200 enemy_ship.bullets = 0 enemy_ship.bullet_radius = 4 enemy_ship.abs_max_bullets = math.floor(math.random(4)) + 1 enemy_ship.target_distance = 80 enemy_ship.shooting_range = 100 enemy_ship.radius = 6 enemy_ship.score = 100 enemy_ship.bullets = 0 enemy_ship.max_bullets = math.floor(math.random(enemy_ship.abs_max_bullets)) + 1 elseif(enemy_ship.ship_type == 3) then enemy_ship.rotation_speed = 0.03 enemy_ship.max_velocity = 1.8 enemy_ship.max_health = 5 enemy_ship.health = 5 enemy_ship.acceleration = 0.15 enemy_ship.target_accuracy =0.15 enemy_ship.bullet_velocity = enemy_ship.max_velocity * 1.5 enemy_ship.bullet_max_age = 180 enemy_ship.bullet_damage = 3 enemy_ship.bullet_tracking = false enemy_ship.bullet_trail = false enemy_ship.bullet_rotation_speed = 0.01 enemy_ship.fire_speed = 16 enemy_ship.bullet_reload = 200 enemy_ship.bullets = 0 enemy_ship.bullet_radius = 5 enemy_ship.abs_max_bullets = math.floor(math.random(6)) + 1 enemy_ship.target_distance = 80 enemy_ship.shooting_range = 100 enemy_ship.radius = 7 enemy_ship.score = 100 enemy_ship.bullets = 0 enemy_ship.max_bullets = math.floor(math.random(enemy_ship.abs_max_bullets)) + 1 elseif(enemy_ship.ship_type == 4) then enemy_ship.rotation_speed = 0.03 enemy_ship.max_velocity = 2 enemy_ship.max_health = 2 enemy_ship.health = 2 enemy_ship.acceleration = 0.2 enemy_ship.target_accuracy =0.15 enemy_ship.bullet_velocity = enemy_ship.max_velocity * 1 enemy_ship.bullet_max_age = 180 enemy_ship.bullet_damage = 3 enemy_ship.bullet_tracking = true enemy_ship.bullet_trail = true enemy_ship.bullet_rotation_speed = 0.014 enemy_ship.fire_speed = 16 enemy_ship.bullet_reload = 200 enemy_ship.bullets = 0 enemy_ship.bullet_radius = 5 enemy_ship.abs_max_bullets = math.floor(math.random(6)) + 1 enemy_ship.target_distance = 80 enemy_ship.shooting_range = 100 enemy_ship.radius = 8 enemy_ship.score = 200 enemy_ship.bullets = 0 enemy_ship.max_bullets = math.floor(math.random(enemy_ship.abs_max_bullets)) + 1 end table.insert(enemies,enemy_ship) end --spawn ememy function update_movement_params() player_ship.movement.dx= player_ship.velocity.magnitude * math.cos(player_ship.velocity.angle) player_ship.movement.dy= player_ship.velocity.magnitude * math.sin(player_ship.velocity.angle) end -- update_movement_params function check_ship_rotation() if(btn(2)) then player_ship.velocity.angle= player_ship.velocity.angle + player_ship_rotation_speed elseif(btn(3)) then player_ship.velocity.angle= player_ship.velocity.angle - player_ship_rotation_speed end player_ship.velocity.angle = wrap_angle(player_ship.velocity.angle) end --check_ship_rotation function check_ship_acceleration() if(btn(0)) then player_ship.velocity.magnitude= player_ship.velocity.magnitude + player_ship_acceleration if (player_ship.velocity.magnitude > player_ship_max_velocity) then player_ship.velocity.magnitude = player_ship_max_velocity end elseif(btn(1)) then player_ship.velocity.magnitude= player_ship.velocity.magnitude - player_ship_acceleration if (player_ship.velocity.magnitude < (-player_ship_max_velocity/2)) then player_ship.velocity.magnitude = (-player_ship_max_velocity/2) end end -- check for stationary if math.abs(player_ship.velocity.magnitude) < (player_ship_acceleration * 0.9) then player_ship.velocity.magnitude = 0 end end --check_ship_acceleration function move_relative_to_player(point) -- moves the point not the ship position point.x=point.x - player_ship.movement.dx point.y=point.y - player_ship.movement.dy return point end -- move_player_ship function move_by_velocity(point, velocity) -- moves the point local dx=velocity.magnitude * math.cos(velocity.angle) local dy=velocity.magnitude * math.sin(velocity.angle) point.x=point.x + dx point.y=point.y + dy return point end function move_enemy_ships() for index, ship in ipairs(enemies) do ship.position = move_relative_to_player(ship.position) end -- for end -- move_enemy_ships function spawn_enemy() if ((#enemies < max_enemies) and (enemy_id_counter < total_enemies)) then enemy_ship = {} enemy_ship.ship_type = 1 + (math.floor(math.random() * (player_level + 1))) if (enemy_ship.ship_type > max_enemy_ship_type) then enemy_ship.ship_type = max_enemy_ship_type end local spawn_angle = math.random() * (2 * math.pi) local spawn_distance = math.floor(math.random(500)) + 1000 local ship_x=spawn_distance * math.cos(spawn_angle) local ship_y=spawn_distance * math.sin(spawn_angle) enemy_ship.id = enemy_id_counter enemy_id_counter = enemy_id_counter + 1 enemy_ship.position = {x=ship_x, y=ship_y} enemy_ship.velocity = {magnitude=0, angle=0} enemy_ship.hit = false enemy_ship.movement_type=0 enemy_ship.timer = 0 if(enemy_ship.ship_type == 1) then enemy_ship.rotation_speed = 0.02 enemy_ship.max_velocity = 1.5 enemy_ship.max_health = 5 enemy_ship.health = 5 enemy_ship.acceleration = 0.05 enemy_ship.target_accuracy =0.2 enemy_ship.bullet_velocity = enemy_ship.max_velocity * 1.5 enemy_ship.bullet_max_age = 120 enemy_ship.bullet_damage = 1 enemy_ship.bullet_tracking = false enemy_ship.bullet_rotation_speed = 0.007 enemy_ship.bullet_trail = false enemy_ship.fire_speed = 32 enemy_ship.bullet_reload = 150 enemy_ship.bullets = 0 enemy_ship.bullet_radius = 4 enemy_ship.abs_max_bullets = 6 enemy_ship.target_distance = 50 enemy_ship.shooting_range = 80 enemy_ship.radius = 5 enemy_ship.score = 50 enemy_ship.bullets = 0 enemy_ship.max_bullets = math.floor(math.random(enemy_ship.abs_max_bullets)) + 1 elseif(enemy_ship.ship_type == 2) then enemy_ship.rotation_speed = 0.03 enemy_ship.max_velocity = 1.7 enemy_ship.max_health = 5 enemy_ship.health = 5 enemy_ship.acceleration = 0.1 enemy_ship.target_accuracy =0.15 enemy_ship.bullet_velocity = enemy_ship.max_velocity * 1 enemy_ship.bullet_max_age = 180 enemy_ship.bullet_damage = 2 enemy_ship.bullet_tracking = true enemy_ship.bullet_trail = true enemy_ship.bullet_rotation_speed = 0.01 enemy_ship.fire_speed = 32 enemy_ship.bullet_reload = 200 enemy_ship.bullets = 0 enemy_ship.bullet_radius = 4 enemy_ship.abs_max_bullets = math.floor(math.random(4)) + 1 enemy_ship.target_distance = 80 enemy_ship.shooting_range = 100 enemy_ship.radius = 6 enemy_ship.score = 100 enemy_ship.bullets = 0 enemy_ship.max_bullets = math.floor(math.random(enemy_ship.abs_max_bullets)) + 1 elseif(enemy_ship.ship_type == 3) then enemy_ship.rotation_speed = 0.03 enemy_ship.max_velocity = 1.8 enemy_ship.max_health = 5 enemy_ship.health = 5 enemy_ship.acceleration = 0.15 enemy_ship.target_accuracy =0.15 enemy_ship.bullet_velocity = enemy_ship.max_velocity * 1.5 enemy_ship.bullet_max_age = 180 enemy_ship.bullet_damage = 3 enemy_ship.bullet_tracking = false enemy_ship.bullet_trail = false enemy_ship.bullet_rotation_speed = 0.01 enemy_ship.fire_speed = 16 enemy_ship.bullet_reload = 200 enemy_ship.bullets = 0 enemy_ship.bullet_radius = 5 enemy_ship.abs_max_bullets = math.floor(math.random(6)) + 1 enemy_ship.target_distance = 80 enemy_ship.shooting_range = 100 enemy_ship.radius = 7 enemy_ship.score = 100 enemy_ship.bullets = 0 enemy_ship.max_bullets = math.floor(math.random(enemy_ship.abs_max_bullets)) + 1 elseif(enemy_ship.ship_type == 4) then enemy_ship.rotation_speed = 0.03 enemy_ship.max_velocity = 2 enemy_ship.max_health = 2 enemy_ship.health = 2 enemy_ship.acceleration = 0.2 enemy_ship.target_accuracy =0.15 enemy_ship.bullet_velocity = enemy_ship.max_velocity * 1 enemy_ship.bullet_max_age = 180 enemy_ship.bullet_damage = 3 enemy_ship.bullet_tracking = true enemy_ship.bullet_trail = true enemy_ship.bullet_rotation_speed = 0.014 enemy_ship.fire_speed = 16 enemy_ship.bullet_reload = 200 enemy_ship.bullets = 0 enemy_ship.bullet_radius = 5 enemy_ship.abs_max_bullets = math.floor(math.random(6)) + 1 enemy_ship.target_distance = 80 enemy_ship.shooting_range = 100 enemy_ship.radius = 8 enemy_ship.score = 200 enemy_ship.bullets = 0 enemy_ship.max_bullets = math.floor(math.random(enemy_ship.abs_max_bullets)) + 1 end table.insert(enemies,enemy_ship) end --if end --spawn ememy function spawn_background() -- initial background in player area background = {} for stars=1, background_max_objects do star_x = math.random(screen_max_x + (2*player_ship_max_velocity)) - player_ship_max_velocity - math.floor(screen_max_x/2) star_y = math.random(screen_max_y + (2*player_ship_max_velocity)) - player_ship_max_velocity - math.floor(screen_max_y/2) generate_background_object(0, star_x, star_y) end --for end -- spawn_background function update_background() local new_object=false for index,object in ipairs(background) do -- update for player moveent object.position = move_relative_to_player(object.position) object.velocity.angle = object.velocity.angle + object.rotation_speed -- wrap objects going out of working background area new_object=false if (object.position.x < -(player_ship_max_velocity + math.floor(screen_max_x/2))) then object.position.x = math.floor(screen_max_x/2) + player_ship_max_velocity new_object=true elseif (object.position.x > (player_ship_max_velocity + math.floor(screen_max_x/2))) then object.position.x = -(math.floor(screen_max_x/2) + player_ship_max_velocity) new_object=true end if (object.position.y < -(player_ship_max_velocity + math.floor(screen_max_y/2))) then object.position.y = math.floor(screen_max_y/2) + player_ship_max_velocity new_object=true elseif (object.position.y > math.floor(screen_max_y/2) + player_ship_max_velocity) then object.position.y = -(math.floor(screen_max_y/2) + player_ship_max_velocity) new_object=true end if (new_object) then object = change_background_object(object) end end -- for end -- update_background function generate_background_object(type, object_x, object_y) if (#background < background_max_objects) then -- make new object object = {} object.type = math.floor(math.random(10)) + 1 if (object.type>2) then object.type = 1 end object.position={} object.position.x = object_x object.position.y = object_y object.velocity={} object.velocity.magnitude=0 object.velocity.angle=0 object.rotation_speed = (math.random(4) - 2) /100 object.age=0 object.colour = math.floor(math.random(15)) + 1 table.insert(background, object) end end -- generate_background_object function change_background_object(object) object.type = math.floor(math.random(10)) + 1 if (object.type>2) then object.type = 1 end object.age=0 object.rotation_speed = (math.random(4) - 2) / 100 object.colour = math.floor(math.random(15)) + 1 return object end -- change_background_object function update_end_game() end -- update_end_game function update_scoreboard() end -- update_scoreboard function draw_start_screen() cls() print("SPACE COMMANDER", 30,30, 6,FALSE,2) spr(16,85,50,-1,3,0,0,2,2) print("PRESS Z TO PLAY", 35,110, 15,FALSE,2) end -- draw_start_screen function draw_playing() cls() --print(player_ship.health, 0,0) --print(angle_to_player.." - "..distance_to_player, 0,8) draw_background() draw_bullets() draw_player_ship() draw_enemy_ships() draw_particles() draw_radar() draw_dashboard() end -- draw_playing function draw_background() for index,object in ipairs(background) do draw_background_object(object) end -- for end -- draw_background function draw_background_object(object) local position = {} local velocity = {} position = translate_position_to_display(object.position) velocity = translate_velocity_to_display(object.velocity) if (object.type == 2) then pix(position.x, position.y, object.colour) pix(position.x+1, position.y, object.colour) pix(position.x, position.y+1, object.colour) pix(position.x+1, position.y+1, object.colour) elseif (object.type==3) then draw_object(10,{x=position.x, y=position.y},velocity.angle) else pix(position.x, position.y, object.colour) end end -- draw_background_object function draw_player_ship() local ship_icon = (player_level*2)+16 if(ship_icon > 22) then ship_icon=22 end spr(ship_icon, screen_offset.x-8, screen_offset.y-8, 0,1,0,0,2,2) end -- draw_player_ship function draw_enemy_ships() local position = {} local velocity = {} for index,ship in ipairs(enemies) do position = translate_position_to_display(ship.position) velocity = translate_velocity_to_display(ship.velocity) ship.screen_position = position if ((math.abs(ship.position.x ) < enemy_min_visible_dist) and (math.abs(ship.position.y) < enemy_min_visible_dist)) then circ(position.x, position.y,ship.radius,0) draw_object(ship.ship_type,position,velocity.angle) end end -- for end --draw_enemy_ships function draw_object(object_type, origin, angle) local object_shape = get_object_shape(object_type) local point={} local last_point={} local rotated_point for index,point in ipairs(object_shape) do -- rotate object point around {0,0} rotated_point = rotate_point({x=0, y=0}, point, angle) point.x = rotated_point.x point.y = rotated_point.y -- move object point to correct screen position point.x = point.x + origin.x point.y = -point.y + origin.y if (point.newline) then last_point = point else line(last_point.x, last_point.y, point.x, point.y, point.colour) last_point=point end end -- for end -- draw_object function get_object_shape(object_type) local object_shape = {} if (object_type == 0) then -- player object_type object_shape = {} table.insert(object_shape, {y=-5,x=-5, newline=true, colour=5}) table.insert(object_shape, {y=-5,x=0, newline=false, colour=5}) table.insert(object_shape, {y=0, x=5, newline=false, colour=5}) table.insert(object_shape, {y=5, x=0, newline=false, colour=5}) table.insert(object_shape, {y=5, x=-5, newline=false, colour=5}) table.insert(object_shape, {y=2, x=-5, newline=false, colour=5}) table.insert(object_shape, {y=2, x=-2, newline=false, colour=5}) table.insert(object_shape, {y=-2, x=-2, newline=false, colour=5}) table.insert(object_shape, {y=-2, x=-5, newline=false, colour=5}) table.insert(object_shape, {y=-5, x=-5, newline=false, colour=5}) table.insert(object_shape, {y=5, x=-5, newline=true, colour=5}) table.insert(object_shape, {y=2, x=-5, newline=false, colour=14}) table.insert(object_shape, {y=-2, x=-5, newline=true, colour=5}) table.insert(object_shape, {y=-5, x=-5, newline=false, colour=14}) elseif(object_type == 1) then -- level 1 ship object_shape = {} table.insert(object_shape, {x=-5, y=-5,newline=true, colour=5}) table.insert(object_shape, {x=0, y=-4,newline=false, colour=5}) table.insert(object_shape, {x=5, y=0, newline=false, colour=5}) table.insert(object_shape, {x=0, y=4, newline=false, colour=5}) table.insert(object_shape, {x=-5, y=5, newline=false, colour=5}) table.insert(object_shape, {x=-4, y=3, newline=false, colour=5}) table.insert(object_shape, {x=-3, y=0, newline=false, colour=5}) table.insert(object_shape, {x=-4, y=-3, newline=false, colour=5}) table.insert(object_shape, {x=-5, y=-5, newline=false, colour=5}) table.insert(object_shape, {x=4, y=0, newline=true, colour=14}) table.insert(object_shape, {x=2, y=1, newline=false, colour=14}) table.insert(object_shape, {x=0, y=0, newline=false, colour=14}) table.insert(object_shape, {x=2, y=-1, newline=false, colour=14}) table.insert(object_shape, {x=4, y=0, newline=false, colour=14}) table.insert(object_shape, {x=0, y=0, newline=false, colour=14}) elseif(object_type == 2) then -- level 2 ship object_shape = {} table.insert(object_shape, {x=-6, y=-6,newline=true, colour=13}) table.insert(object_shape, {x=3, y=-6,newline=false, colour=13}) table.insert(object_shape, {x=-2, y=-4, newline=false, colour=13}) table.insert(object_shape, {x=-3, y=-2, newline=false, colour=13}) table.insert(object_shape, {x=4, y=2, newline=false, colour=13}) table.insert(object_shape, {x=6, y=0, newline=false, colour=13}) table.insert(object_shape, {x=4, y=-2, newline=false, colour=13}) table.insert(object_shape, {x=-3, y=2, newline=false, colour=13}) table.insert(object_shape, {x=-2, y=4, newline=false, colour=13}) table.insert(object_shape, {x=3, y=6, newline=false, colour=13}) table.insert(object_shape, {x=-6, y=6, newline=false, colour=13}) table.insert(object_shape, {x=-4, y=0, newline=false, colour=13}) table.insert(object_shape, {x=-6, y=-6, newline=false, colour=13}) table.insert(object_shape, {x=5, y=0, newline=true, colour=9}) table.insert(object_shape, {x=3, y=1, newline=false, colour=9}) table.insert(object_shape, {x=1, y=0, newline=false, colour=9}) table.insert(object_shape, {x=3, y=-1, newline=false, colour=9}) table.insert(object_shape, {x=5, y=0, newline=false, colour=9}) table.insert(object_shape, {x=1, y=0, newline=false, colour=9}) elseif(object_type == 3) then -- level 3 ship object_shape = {} table.insert(object_shape, {x=-6, y=-0,newline=true, colour=6}) table.insert(object_shape, {x=8, y=-3,newline=false, colour=6}) table.insert(object_shape, {x=6, y=0, newline=false, colour=6}) table.insert(object_shape, {x=8, y=3, newline=false, colour=6}) table.insert(object_shape, {x=-6, y=0, newline=false, colour=6}) table.insert(object_shape, {x=-4, y=1, newline=true, colour=6}) table.insert(object_shape, {x=-2, y=6, newline=false, colour=6}) table.insert(object_shape, {x=2, y=2, newline=false, colour=6}) table.insert(object_shape, {x=-4, y=1, newline=true, colour=6}) table.insert(object_shape, {x=0, y=3, newline=false, colour=6}) table.insert(object_shape, {x=-4, y=1, newline=true, colour=6}) table.insert(object_shape, {x=-2, y=-6, newline=false, colour=6}) table.insert(object_shape, {x=2, y=-2, newline=false, colour=6}) table.insert(object_shape, {x=-4, y=-1, newline=true, colour=6}) table.insert(object_shape, {x=0, y=-3, newline=false, colour=6}) table.insert(object_shape, {x=5, y=0, newline=true, colour=14}) table.insert(object_shape, {x=3, y=1, newline=false, colour=14}) table.insert(object_shape, {x=1, y=0, newline=false, colour=14}) table.insert(object_shape, {x=3, y=-1, newline=false, colour=14}) table.insert(object_shape, {x=5, y=0, newline=false, colour=14}) table.insert(object_shape, {x=1, y=0, newline=false, colour=14}) elseif(object_type == 4) then -- level 4 ship object_shape = {} table.insert(object_shape, {x=-7, y=0,newline=true, colour=8}) table.insert(object_shape, {x=-4, y=1,newline=false, colour=8}) table.insert(object_shape, {x=-2, y=4,newline=false, colour=8}) table.insert(object_shape, {x=-4, y=4,newline=false, colour=8}) table.insert(object_shape, {x=-6, y=6,newline=false, colour=8}) table.insert(object_shape, {x=-7, y=9,newline=false, colour=8}) table.insert(object_shape, {x=-1, y=9,newline=false, colour=8}) table.insert(object_shape, {x=2, y=7,newline=false, colour=8}) table.insert(object_shape, {x=5, y=4,newline=false, colour=8}) table.insert(object_shape, {x=7, y=0,newline=false, colour=8}) table.insert(object_shape, {x=5, y=-4,newline=false, colour=8}) table.insert(object_shape, {x=2, y=-7,newline=false, colour=8}) table.insert(object_shape, {x=-1, y=-9,newline=false, colour=8}) table.insert(object_shape, {x=-7, y=-9,newline=false, colour=8}) table.insert(object_shape, {x=-6, y=-6,newline=false, colour=8}) table.insert(object_shape, {x=-4, y=-4,newline=false, colour=8}) table.insert(object_shape, {x=-2, y=-4,newline=false, colour=8}) table.insert(object_shape, {x=-4, y=-1,newline=false, colour=8}) table.insert(object_shape, {x=-7, y=-0,newline=false, colour=8}) table.insert(object_shape, {x=5, y=4,newline=true, colour=8}) table.insert(object_shape, {x=3, y=1,newline=false, colour=8}) table.insert(object_shape, {x=2, y=0,newline=false, colour=8}) table.insert(object_shape, {x=3, y=-1,newline=false, colour=8}) table.insert(object_shape, {x=5, y=-4,newline=false, colour=8}) elseif (object_type == 10) then --background star object_shape = {} table.insert(object_shape, {y=0,x=1, newline=true, colour=10}) table.insert(object_shape, {y=0,x=-1, newline=false, colour=math.floor(math.random(15)) + 1}) table.insert(object_shape, {y=1, x=0, newline=true, colour=10}) table.insert(object_shape, {y=-1, x=0, newline=false, colour=math.floor(math.random(15)) + 1}) elseif (object_type == 20) then -- player bullet object_shape = {} table.insert(object_shape, {x=0,y=0, newline=true, colour=10}) table.insert(object_shape, {x=5,y=0, newline=false, colour=10}) elseif (object_type == 21) then -- player bullet object_shape = {} table.insert(object_shape, {x=0,y=0, newline=true, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=3,y=0, newline=false, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=0,y=0, newline=true, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=-3,y=0, newline=false, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=0,y=0, newline=true, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=0,y=3, newline=false, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=0,y=0, newline=true, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=0,y=-3, newline=false, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=0,y=0, newline=true, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=-2,y=-2, newline=false, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=0,y=0, newline=true, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=2,y=-2, newline=false, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=0,y=0, newline=true, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=2,y=2, newline=false, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=0,y=0, newline=true, colour=math.floor(math.random(4))+7}) table.insert(object_shape, {x=-2,y=2, newline=false, colour=math.floor(math.random(4))+7}) elseif (object_type == 30) then -- enemy bullet type1 object_shape = {} table.insert(object_shape, {x=0,y=0, newline=true, colour=9}) table.insert(object_shape, {x=5,y=0, newline=false, colour=9}) elseif (object_type == 31) then -- enemy bullet type2 object_shape = {} table.insert(object_shape, {x=0,y=0, newline=true, colour=10}) table.insert(object_shape, {x=5,y=0, newline=false, colour=10}) table.insert(object_shape, {x=0,y=1, newline=true, colour=10}) table.insert(object_shape, {x=0,y=-1, newline=false, colour=10}) table.insert(object_shape, {x=3,y=1, newline=true, colour=10}) table.insert(object_shape, {x=3,y=-1, newline=false, colour=10}) elseif (object_type == 32) then -- enemy bullet type3 object_shape = {} table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=3,y=0, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=-3,y=0, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=3, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=-3, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=-2,y=-2, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=2,y=-2, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=2,y=2, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=-2,y=2, newline=false, colour=get_fire_colour()}) elseif (object_type == 33) then -- enemy bullet type4 object_shape = {} table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=3,y=0, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=-3,y=0, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=3, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=-3, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=-2,y=-2, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=2,y=-2, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=2,y=2, newline=false, colour=get_fire_colour()}) table.insert(object_shape, {x=0,y=0, newline=true, colour=get_fire_colour()}) table.insert(object_shape, {x=-2,y=2, newline=false, colour=get_fire_colour()}) end return object_shape end -- get_object_shape function get_fire_colour() -- returns a fire colour local colours = {6,8,12,14,15} return colours[math.random(#colours)] end -- get_fire_colour function rotate_point(origin, point, angle) local transposed_point = {} transposed_point.x = point.x - origin.x transposed_point.y = point.y - origin.y local rotated_point = {} rotated_point.x = (transposed_point.x * math.cos(angle)) - (transposed_point.y * math.sin(angle)) rotated_point.y = (transposed_point.y * math.cos(angle)) + (transposed_point.x * math.sin(angle)) -- update provided point rotated_point.x = rotated_point.x + origin.x rotated_point.y = rotated_point.y + origin.y return rotated_point end -- rotate_point function translate_position_to_display(position) local new_position = {} local adjusted_angle = (math.pi/2) - player_ship.velocity.angle adjusted_angle = wrap_angle(adjusted_angle) new_position = rotate_point({x=0,y=0}, position, adjusted_angle) new_position.x = new_position.x + screen_offset.x new_position.y = -new_position.y + screen_offset.y return new_position end -- translate_position_to_display function translate_velocity_to_display(velocity) local new_velocity={} new_velocity.angle = velocity.angle + (math.pi/2) - player_ship.velocity.angle new_velocity.angle = wrap_angle(new_velocity.angle) new_velocity.magnitude = velocity.magnitude return new_velocity end -- translate_velocity_to_display -- add two angle / magnitude vectors function add_velocities(v1, v2) local dx1=v1.magnitude * math.cos(v1.angle) local dy1=v1.magnitude * math.sin(v1.angle) local dx2=v2.magnitude * math.cos(v2.angle) local dy2=v2.magnitude * math.sin(v2.angle) local dx = dx1 + dx2 local dy = dy1 + dy2 local velocity = {} velocity.dx = dx velocity.dy = dy velocity.angle = math.atan2(dy, dx) velocity.magnitude = math.sqrt((dx*dx) + (dy*dy)) return velocity end function get_angle_to_target(subject, target) local dx = target.position.x-subject.position.x local dy = target.position.y-subject.position.y local angle_to_target = math.atan2(dy, dx) angle_to_target = wrap_angle(angle_to_target) return angle_to_target end --get_angle_to_target function wrap_angle(angle) local new_angle = angle if (new_angle > (2*math.pi)) then new_angle = new_angle - (2*math.pi) elseif(new_angle < 0) then new_angle = new_angle + (2*math.pi) end --if return new_angle end -- wrap_angle function add_angles(angle1, angle2) local new_angle = angle1 + angle2 new_angle = wrap_angle(new_angle) return new_angle end -- add_angles function get_angle_inc(target_angle, current_angle, target_accuracy) local increment = 0 local angle_diff = target_angle - current_angle if (angle_diff < 0) then angle_diff = (2*math.pi) + angle_diff end -- if if (math.abs(angle_diff) < target_accuracy) then increment = 0 elseif (angle_diff > math.pi) then increment = -1 else increment=1 end -- if return increment end -- get_angle_inc -- first run code -- run init functions to set up game init_game_state() init_variables(true)